1.5 Static Members
In some cases, certain members should belong only to the class; that is, they should not be part of any instance of the class. Such members are called static members. Fields and methods that are static members are easily distinguishable in a class declaration as they must always be declared with the keyword static.
Figure 1.3 shows the class diagram for the class Point2D. It has been augmented by three static members, whose names are underlined to distinguish them from instance members. The augmented declaration of the Point2D class is given in Example 1.2.
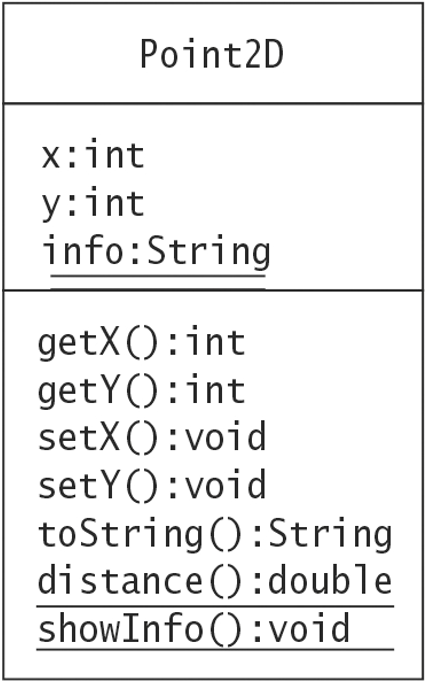
Figure 1.3 Class Diagram Showing Static Members of a Class
In Example 1.2, the field info at (1) is declared as a static variable. This field has information about the purpose of the class that the class can share with its clients. A static variable belongs to the class, rather than to any specific object of the class. It will be allocated in the class and initialized to the string specified in its declaration when the class is loaded. Declaring the info field as static makes sense, as it is unnecessary that every object of the class Point2D should have a copy of this information.
private static String info = “A point represented by (x,y)-coordinates.”;
In Example 1.2, the two methods distance() and showInfo() at (5) are static methods belonging to the class. Both are declared with the keyword static. The static method distance() calculates and returns the distance between two points passed as arguments to the method. The static method showInfo() prints the string with the information referenced by the static variable info. These methods belong to the class, rather than to any specific objects of the class.
Clients can access static members in the class by using the class name. The following code invokes the static method distance() in the class Point2D:
double d = Point2D.distance(p1, p2); // Class name to invoke static method
Static members can also be accessed via object references, although doing so is not encouraged:
p1.showInfo(); // Reference invokes static method
Static members in a class can be accessed both by the class name and via object references, but instance members can be accessed only by object references.
Example 1.2 Static Members in Class Declaration
Click here to view code image
// File: Point2D.java
public class Point2D { // Class name
// Class Member Declarations
// Static variable: (1)
private static String info = “A 2D point represented by (x,y)-coordinates.”;
// Instance variables: (2)
private int x;
private int y;
// Constructor: (3)
public Point2D(int xCoord, int yCoord) {
x = xCoord;
y = yCoord;
}
// Instance methods: (4)
public int getX() { return x; }
public int getY() { return y; }
public void setX(int xCoord) { x = xCoord; }
public void setY(int yCoord) { y = yCoord; }
public String toString() { return “(” + x + “,” + y + “)”; } // Format: (x,y)
// Static methods: (5)
public static double distance(Point2D p1, Point2D p2) {
int xDiff = p1.x – p2.x;
int yDiff = p1.y – p2.y;
return Math.sqrt(xDiff*xDiff + yDiff*yDiff);
}
public static void showInfo() { System.out.println(info); }
}
Figure 1.4 shows the classification of the members in the class Point2D, using the terminology we have introduced so far. Table 1.1 provides a summary of the terminology used in defining members of a class.
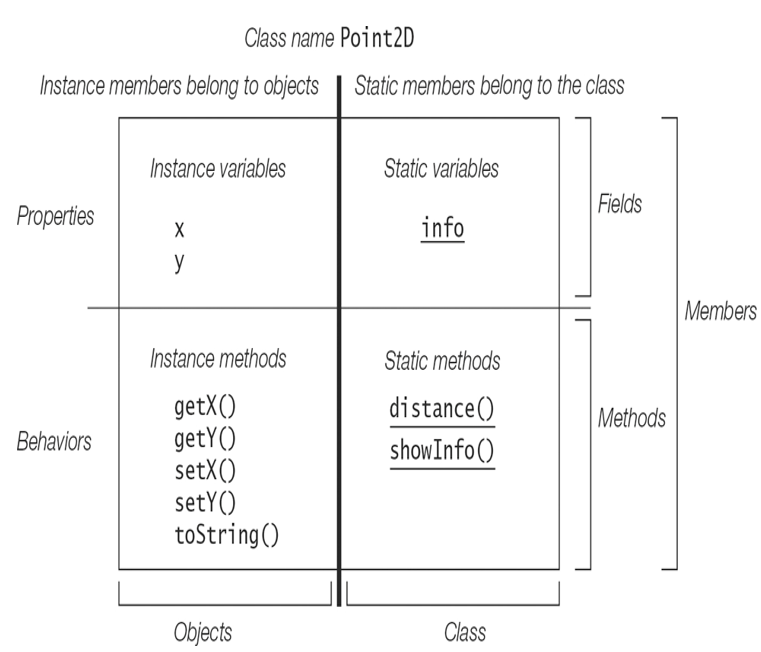
Figure 1.4 Members of a Class
Table 1.1 Terminology for Class Members
Instance members | The instance variables and instance methods of an object. They can be accessed or invoked only through an object reference. |
Instance variable | A field that is allocated when the class is instantiated (i.e., when an object of the class is created). Also called a non-static field or just a field when the context is obvious. |
Instance method | A method that belongs to an instance of the class. Objects of the same class share its implementation. |
Static members | The static variables and static methods of a class. They can be accessed or invoked either by using the class name or through an object reference. |
Static variable | A field that is allocated when the class is loaded. It belongs to the class, and not to any specific object of the class. Also called a static field or a class variable. |
Static method | A method that belongs to the class, and not to any object of the class. Also called a class method. |